Mastering the J Hook in STL: A Beginner's Guide
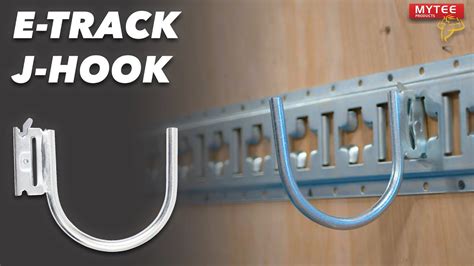
Understanding the J Hook in STL
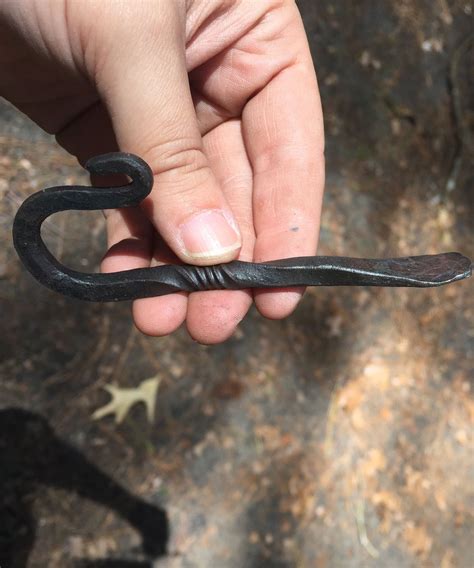
The J hook is a fundamental technique in the STL (Standard Template Library) that allows for efficient and flexible container manipulation. Despite its importance, many programmers struggle to grasp the concept, leading to inefficient code and unnecessary complexity. In this guide, we’ll delve into the world of J hooks, exploring what they are, how they work, and how to master them.
What is a J Hook?
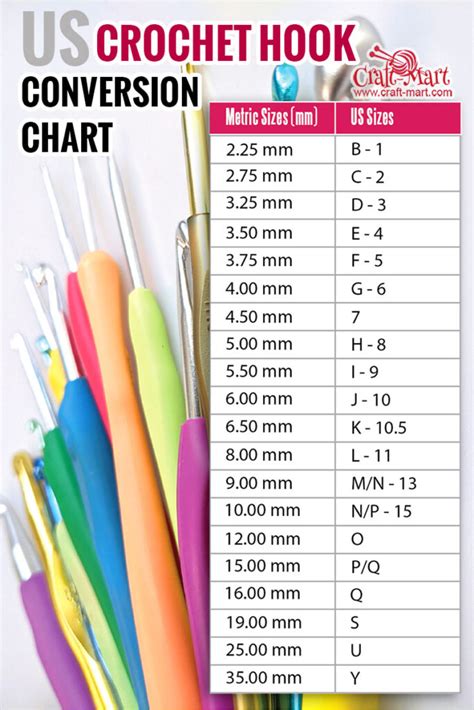
A J hook, also known as a “ iterator hook,” is a mechanism that enables the connection of two or more containers in a way that allows for seamless iteration and manipulation of their elements. It’s a crucial component in STL’s container-adaptor framework, which provides a way to adapt and transform containers to meet specific requirements.
Think of a J hook as a “ bridge” that links two containers, enabling you to iterate over their combined elements as if they were a single container. This allows for efficient and flexible manipulation of container data, making it a powerful tool in the STL arsenal.
How Does a J Hook Work?
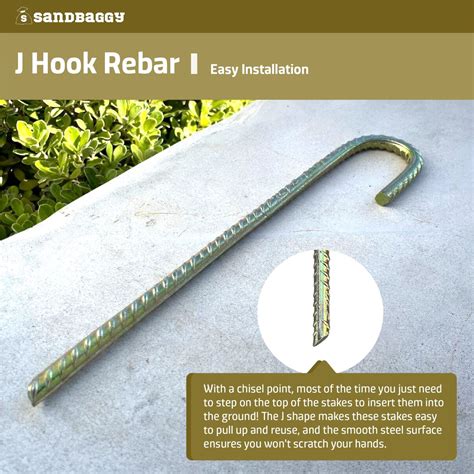
To understand how a J hook works, let’s consider a simple example. Suppose we have two containers, container_a
and container_b
, each containing a set of elements.
// container_a
std::vector<int> container_a = {1, 2, 3};
// container_b
std::vector<int> container_b = {4, 5, 6};
We want to iterate over the combined elements of both containers as if they were a single container. This is where the J hook comes in.
// create a J hook
std::joint_iterator<int> joint_iterator(container_a.begin(), container_a.end(), container_b.begin(), container_b.end());
// iterate over the combined elements
for (; joint_iterator!= joint_iterator.end(); ++joint_iterator) {
std::cout << *joint_iterator << " ";
}
Output:
1 2 3 4 5 6
As you can see, the J hook allows us to iterate over the combined elements of both containers seamlessly.
Mastering the J Hook: Tips and Tricks
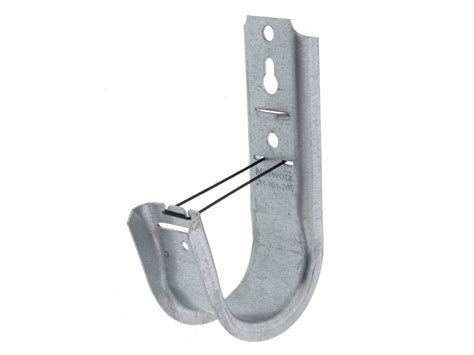
Now that you understand the basics of J hooks, here are some tips and tricks to help you master this powerful technique:
- Use the
std::joint_iterator
class: Thestd::joint_iterator
class is a special iterator designed specifically for J hooks. It provides a convenient way to create and manage J hooks. - Pass the correct iterators: When creating a J hook, make sure to pass the correct iterators to the
std::joint_iterator
constructor. This includes the begin and end iterators for both containers. - Use the
joint_iterator
in algorithms: J hooks can be used with STL algorithms, making it easy to perform complex operations on combined containers. - Be mindful of iterator invalidation: When using J hooks, be aware of iterator invalidation, which can occur when the underlying containers are modified.
🔍 Note: When using J hooks with algorithms, make sure to pass the correct iterators to avoid iterator invalidation.
Common Use Cases for J Hooks

J hooks have a wide range of applications in STL programming. Here are some common use cases:
- Merging containers: J hooks can be used to merge two or more containers into a single container, allowing for efficient iteration and manipulation of the combined elements.
- Splitting containers: J hooks can also be used to split a single container into multiple containers, making it easy to process and manipulate subsets of data.
- Implementing container adaptors: J hooks are a key component in implementing container adaptors, which provide a way to transform and adapt containers to meet specific requirements.
Conclusion
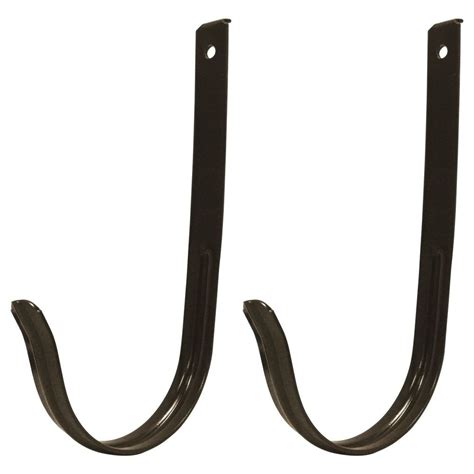
Mastering the J hook is an essential skill for any STL programmer. By understanding how J hooks work and how to use them effectively, you can write more efficient, flexible, and powerful code. Remember to use the std::joint_iterator
class, pass the correct iterators, and be mindful of iterator invalidation. With practice and experience, you’ll become proficient in using J hooks to solve complex problems in STL programming.
What is a J hook in STL?

+
A J hook is a mechanism that enables the connection of two or more containers in a way that allows for seamless iteration and manipulation of their elements.
How do I create a J hook in STL?
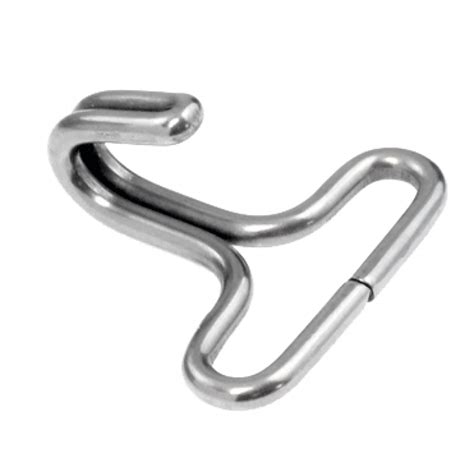
+
You can create a J hook using the std::joint_iterator
class, passing the begin and end iterators for both containers to the constructor.
What are some common use cases for J hooks in STL?

+
J hooks can be used for merging containers, splitting containers, and implementing container adaptors.