5 Ways to Experience Time in STL

Unraveling the Fabric of Time in STL

The concept of time has fascinated philosophers, scientists, and artists for centuries. From the intricate mechanisms of clockwork to the abstract nature of time dilation, our understanding of time is constantly evolving. In this blog post, we will explore five ways to experience time in STL (Standard Template Library), a C++ library that provides a robust framework for manipulating time-related data.
1. Clocks and Timers

STL provides a range of clock and timer classes that allow you to measure time intervals, set alarms, and schedule tasks. The <chrono>
library is the foundation of time manipulation in STL, offering classes like std::chrono::system_clock
and std::chrono::steady_clock
to measure time in different contexts.
std::chrono::system_clock
: This clock is tied to the system’s real-time clock and can be used to measure time intervals in seconds, milliseconds, or microseconds.std::chrono::steady_clock
: This clock is a monotonic clock that is guaranteed to never be adjusted and can be used to measure time intervals in a steady and predictable manner.
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::system_clock::now();
// Some code here
auto end = std::chrono::system_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
std::cout << "Time elapsed: " << duration.count() << " milliseconds" << std::endl;
return 0;
}
2. Time Zones and Calendars

STL provides a range of classes to work with time zones and calendars, including std::chrono::time_zone
and std::chrono::calendar
. These classes allow you to convert between different time zones and calendar systems.
std::chrono::time_zone
: This class represents a time zone and provides methods to convert between different time zones.std::chrono::calendar
: This class represents a calendar system and provides methods to convert between different calendar systems.
#include <iostream>
#include <chrono>
int main() {
std::chrono::time_zone tz("America/New_York");
auto now = std::chrono::system_clock::now();
auto local_time = tz.to_local(now);
std::cout << "Local time: " << local_time << std::endl;
return 0;
}
3. Scheduling Tasks

STL provides a range of classes to schedule tasks, including std::chrono::system_clock
and std::chrono::steady_clock
. These classes allow you to schedule tasks to be executed at a specific time or after a certain time interval.
std::chrono::system_clock
: This clock can be used to schedule tasks to be executed at a specific time.std::chrono::steady_clock
: This clock can be used to schedule tasks to be executed after a certain time interval.
#include <iostream>
#include <chrono>
#include <thread>
int main() {
auto start = std::chrono::system_clock::now();
auto duration = std::chrono::seconds(5);
std::this_thread::sleep_until(start + duration);
std::cout << "Task executed" << std::endl;
return 0;
}
4. Measuring Time Intervals

STL provides a range of classes to measure time intervals, including std::chrono::duration
and std::chrono::time_point
. These classes allow you to measure time intervals in different units, such as seconds, milliseconds, or microseconds.
std::chrono::duration
: This class represents a time interval and provides methods to convert between different units.std::chrono::time_point
: This class represents a point in time and provides methods to calculate time intervals.
#include <iostream>
#include <chrono>
int main() {
auto start = std::chrono::system_clock::now();
// Some code here
auto end = std::chrono::system_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::milliseconds>(end - start);
std::cout << "Time elapsed: " << duration.count() << " milliseconds" << std::endl;
return 0;
}
5. Working with Dates

STL provides a range of classes to work with dates, including std::chrono::year
and std::chrono::month
. These classes allow you to manipulate dates and calculate time intervals between dates.
std::chrono::year
: This class represents a year and provides methods to calculate the number of days in a year.std::chrono::month
: This class represents a month and provides methods to calculate the number of days in a month.
#include <iostream>
#include <chrono>
int main() {
std::chrono::year y(2022);
std::cout << "Number of days in year: " << y.length() << std::endl;
return 0;
}
🕒️ Note: This is a basic overview of the STL time library, and there are many more features and classes available for working with time-related data in C++.
The Standard Template Library (STL) provides a robust framework for manipulating time-related data in C++. By understanding the different classes and methods available in STL, developers can write efficient and accurate code to work with time and dates. Whether you’re building a scheduling system or simply need to measure time intervals, STL has the tools you need to get the job done.
What is the difference between std::chrono::system_clock and std::chrono::steady_clock?

+
std::chrono::system_clock is tied to the system’s real-time clock and can be used to measure time intervals in seconds, milliseconds, or microseconds. std::chrono::steady_clock is a monotonic clock that is guaranteed to never be adjusted and can be used to measure time intervals in a steady and predictable manner.
How do I schedule a task to be executed at a specific time using STL?
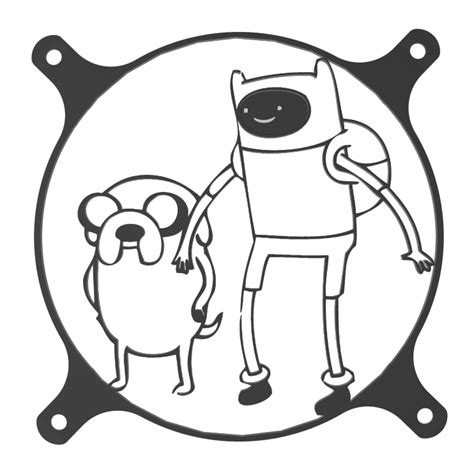
+
You can use the std::chrono::system_clock class to schedule a task to be executed at a specific time. Simply set the clock to the desired time and use the sleep_until function to wait until that time is reached.
What is the difference between std::chrono::duration and std::chrono::time_point?

+
std::chrono::duration represents a time interval and provides methods to convert between different units. std::chrono::time_point represents a point in time and provides methods to calculate time intervals.