5 Types of Special Operators You Should Know
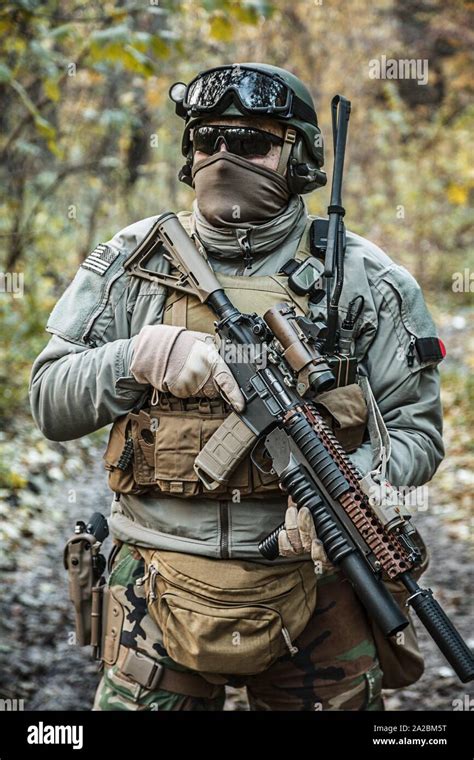
Introduction to Special Operators
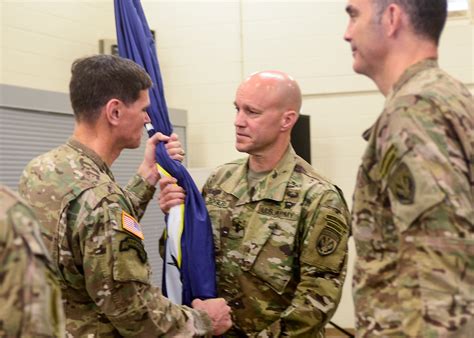
In programming, operators are symbols used to perform operations on variables and values. While most programmers are familiar with arithmetic, comparison, and logical operators, special operators are often overlooked, yet they are incredibly useful in specific situations. In this article, we will explore five types of special operators that you should know, their uses, and examples of how to use them.
1. Ternary Operator (Conditional Operator)

The ternary operator, also known as the conditional operator, is a shorthand way to write simple if-else statements. It consists of three parts: a condition, a value if the condition is true, and a value if the condition is false.
Syntax: condition? value_if_true : value_if_false
Example:
let age = 25;
let status = age >= 18? "Adult" : "Minor";
console.log(status); // Output: "Adult"
The ternary operator is useful when you need to assign a value to a variable based on a condition.
2. Spread Operator

The spread operator is used to expand an array or an object into individual elements. It is denoted by three dots (...
).
Syntax: [...array]
or {...object}
Example:
let numbers = [1, 2, 3];
let moreNumbers = [...numbers, 4, 5, 6];
console.log(moreNumbers); // Output: [1, 2, 3, 4, 5, 6]
The spread operator is useful when you need to merge arrays or objects.
3. Rest Operator

The rest operator is similar to the spread operator, but it is used to collect remaining elements into an array. It is also denoted by three dots (...
).
Syntax: function(...args)
Example:
function sum(...args) {
let total = 0;
for (let num of args) {
total += num;
}
return total;
}
console.log(sum(1, 2, 3, 4, 5)); // Output: 15
The rest operator is useful when you need to create a function that accepts a variable number of arguments.
4. Optional Chaining Operator

The optional chaining operator is used to access nested properties of an object without causing a null pointer exception. It is denoted by a question mark (?
) followed by a dot (.
).
Syntax: object?.property
Example:
let person = {
name: "John",
address: {
street: "123 Main St",
city: "Anytown"
}
};
console.log(person.address?.city); // Output: "Anytown"
The optional chaining operator is useful when you need to access nested properties of an object without knowing if they exist.
5. Nullish Coalescing Operator
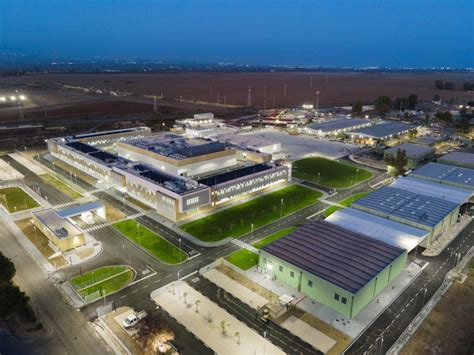
The nullish coalescing operator is used to return the first operand if it is not null or undefined, and the second operand if it is. It is denoted by two question marks (??
).
Syntax: operand1?? operand2
Example:
let name = null;
let fullName = name?? "Unknown";
console.log(fullName); // Output: "Unknown"
The nullish coalescing operator is useful when you need to provide a default value for a variable that might be null or undefined.
📝 Note: The nullish coalescing operator is not the same as the OR operator (`||`). The OR operator returns the first truthy value, while the nullish coalescing operator returns the first non-nullish value.
In conclusion, special operators can simplify your code and make it more readable. By using the ternary operator, spread operator, rest operator, optional chaining operator, and nullish coalescing operator, you can write more concise and efficient code.
What is the difference between the spread operator and the rest operator?

+
The spread operator is used to expand an array or an object into individual elements, while the rest operator is used to collect remaining elements into an array.
When should I use the optional chaining operator?

+
You should use the optional chaining operator when you need to access nested properties of an object without causing a null pointer exception.
What is the difference between the nullish coalescing operator and the OR operator?
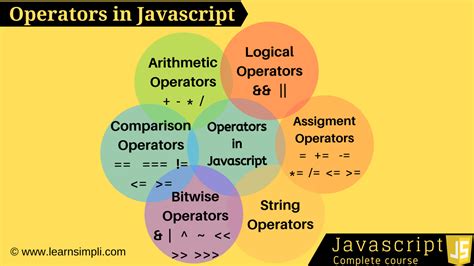
+
The nullish coalescing operator returns the first non-nullish value, while the OR operator returns the first truthy value.
Related Terms:
- Special Operations Command
- NATO Special Forces
- List of Special Forces
- Special Forces Operator salary
- Marine
- NATO Base